1.在 DLL這裡做修改
MyDLL.h
#pragma once
#ifdef MYDLL_EXPORTS
#define MYDLL_API __declspec(dllexport)
#else
#define MYDLL_API __declspec(dllimport)
#endif
using namespace std;
class MYDLL_API ADD_Control {
public:
ADD_Control();
int Add_test(int i,int j);
int ADD_ID = 0;
private:
};
拉出一個指標,用指標來接收訊息
直接加入一個CLASS的 new 跟 Delet 來建立跟刪除
MyDLL.cpp
#include "pch.h"
#include <cmath>
#include <iostream>
#include "MyDLL.h"
ADD_Control::ADD_Control()
{
std::cout << "Hello ADD";
}
int ADD_Control::Add_test(int i, int j)
{
return i + j;
}
//////////////////////////////////////////////////////
extern "C" MYDLL_API ADD_Control *ADD_Ptr_Csharp = NULL;
extern "C" MYDLL_API ADD_Control * Create_Class();
extern ADD_Control* Create_Class()
{
return new ADD_Control();
}
extern "C" MYDLL_API ADD_Control * Delete_Class(ADD_Control * ADD_Csharp);
extern ADD_Control* Delete_Class(ADD_Control* ADD_Csharp)
{
delete ADD_Csharp;
ADD_Csharp = NULL;
return ADD_Csharp;
}
extern "C" MYDLL_API int ADD_test1(ADD_Control * ADD_Csharp, int i, int j);
extern int ADD_test1(ADD_Control* ADD_Csharp, int i, int j)
{
return ADD_Csharp->Add_test(i, j);
}
extern "C" MYDLL_API int ADD_test2(ADD_Control * ADD_Csharp);
extern int ADD_test2(ADD_Control* ADD_Csharp)
{
ADD_Csharp->ADD_ID = 5;
return ADD_Csharp->ADD_ID;
}
2. 最後一樣用PInvoke去接,但是要用指標去接Class
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Runtime.InteropServices;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
m_ADD_ptr = Create_Class();
}
private void button2_Click(object sender, EventArgs e)
{
m_ADD_ptr = Delete_Class(m_ADD_ptr);
}
private void button3_Click(object sender, EventArgs e)
{
button3.Text = ADD_test1(m_ADD_ptr, 1, 2).ToString();
}
private void button4_Click(object sender, EventArgs e)
{
button4.Text = ADD_test2(m_ADD_ptr).ToString();
}
}
}
namespace WindowsFormsApp1
{
public partial class Form1
{
private IntPtr m_ADD_ptr;
[DllImport("MyDLL.dll", EntryPoint = "Create_Class", CallingConvention = CallingConvention.Cdecl)]
private static extern IntPtr Create_Class();
[DllImport("MyDLL.dll", EntryPoint = "Delete_Class", CallingConvention = CallingConvention.Cdecl)]
private static extern IntPtr Delete_Class(IntPtr ADD_ptr);
[DllImport("MyDLL.dll", EntryPoint = "ADD_test1", CallingConvention = CallingConvention.Cdecl)]
private static extern int ADD_test1(IntPtr ADD_ptr, int i, int j);
[DllImport("MyDLL.dll", EntryPoint = "ADD_test2", CallingConvention = CallingConvention.Cdecl)]
private static extern int ADD_test2(IntPtr ADD_ptr);
}
}
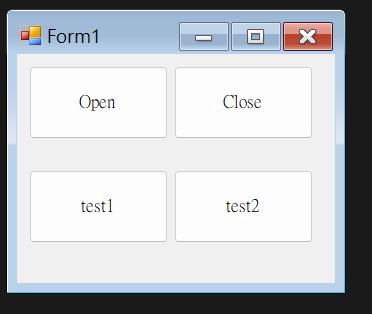