[.NET] [C#] [JSON.NET]
使用JSON.NET
SerializeObject()將物件與Dataset序列化(Serialize)為JSON
DeserializeObject()將Json反序列化(Deserialize)為物件
JSON
JSON是一種簡單輕量的資料交換格式。可用 陣列 [ ] 與 物件 { } 來寫入資料,資料的名稱與值;name / value 成對,名稱與值的中間以 : 隔開,如下:
[
{
"Name": "Jason",
"Age": 18,
"Gender": "male"
},
{
"Name": "Wendy",
"Age": 30,
"Gender": "female"
},
{
"Name": "Alex",
"Age": 50,
"Gender": "male"
},
{
"Name": "Amanda",
"Age": 21,
"Gender": "female"
}
]
JSON.NET
JSON.NET 官網
JSON.NET 說明
到官網下載JSON.NET,將Newtonsoft.Json.dll加入至參考。
加入using,便可使用JSON.NET。
using Newtonsoft.Json;
Serializing序列化
SeserializeObject(): 序列化成JSON。
序列化成物件
建立Introduction類別與Introduction物件並給予值。
protected void Page_Load(object sender, EventArgs e)
{
Introduction Introduction = new Introduction
{
Name = "Berry",
Age = 18,
Marry = false,
Habit = new List<string>
{
"Sing",
"Dance",
"Code",
"Sleep"
}
};
}
public class Introduction
{
public string Name { get; set; } //名稱
public int Age { get; set; } //年紀
public bool Marry { get; set; } //結婚
public List<string> Habit { get; set; } //興趣
}
加入JsonConvert.SerializeObject(物件),將物件序列化成JSON格式。
protected void Page_Load(object sender, EventArgs e)
{
Introduction Introduction = new Introduction
{
Name = "Berry",
Age = 18,
Marry = false,
Habit = new List<string>
{
"Sing",
"Dance",
"Code",
"Sleep"
}
};
//轉成JSON格式
string json = JsonConvert.SerializeObject(Introduction);
//顯示
Response.Write(json);
}
public class Introduction
{
public string Name { get; set; } //名稱
public int Age { get; set; } //年紀
public bool Marry { get; set; } //結婚
public List<string> Habit { get; set; } //興趣
}
最後顯示畫面。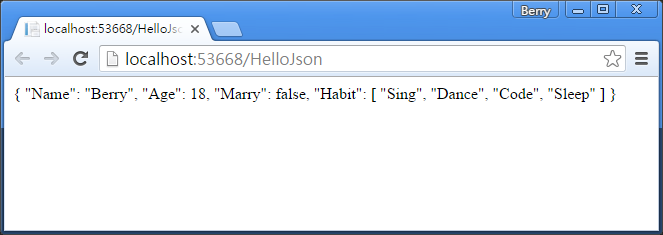
轉換出來的JSON格式可以貼在 http://jsoneditoronline.org/ ,看看轉出的資料是否正確。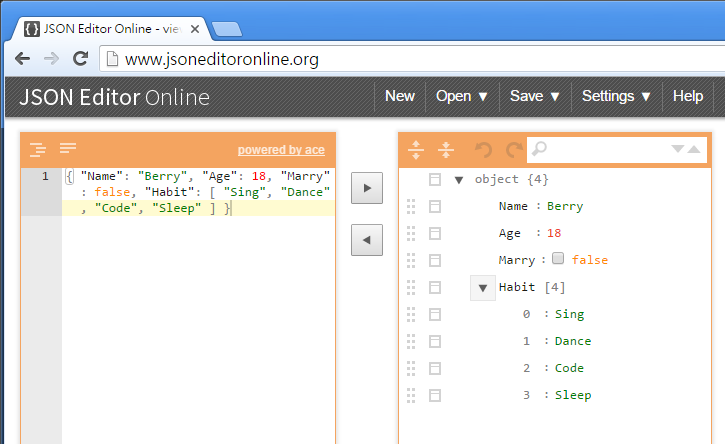
序列化成DataSet
引用System.Data。
using System.Data;
JsonConvert.SerializeObject(DataSet) ,將DataSet序列化成JSON格式。
protected void Page_Load(object sender, EventArgs e)
{
DataSet dataSet = new DataSet("dataSet"); //建立DataSet
DataTable table = new DataTable(); //建立DataTable
DataColumn idColumn = new DataColumn("id", typeof(int)); //建立id欄
DataColumn itemColumn = new DataColumn("item"); //建立item欄
//DataTable加入欄位
table.Columns.Add(idColumn);
table.Columns.Add(itemColumn);
//DataSet加入Table
dataSet.Tables.Add(table);
//跑回圈產生資料
for (int i = 1; i < 5; i++)
{
DataRow newRow = table.NewRow();
newRow["id"] = i;
newRow["item"] = "這是第" + i + "個項目";
table.Rows.Add(newRow);
}
//轉成JSON格式
string json = JsonConvert.SerializeObject(dataSet);
//顯示
Response.Write(json);
}
最後顯示畫面。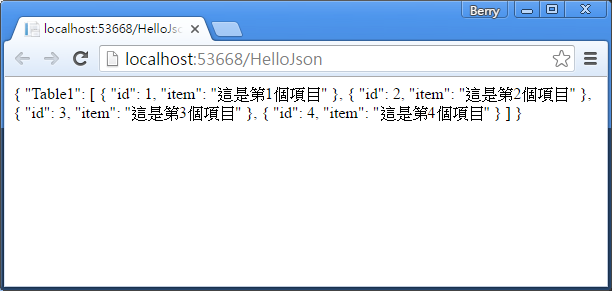
Deserialize反序列化
DeserializeObject(): 將JSON格式轉換成物件。
反序列化成物件
JsonConvert.DeserializeObject<類別>("字串"),<>中定義類別,將JSON字串反序列化成物件。
protected void Page_Load(object sender, EventArgs e)
{
//JSON字串
string Json="{ 'Name': 'Berry', 'Age': 18, 'Marry': false, 'Habit': [ 'Sing','Dance','Code','Sleep' ] }";
//轉成物件
Introduction Introduction = JsonConvert.DeserializeObject<Introduction>(Json);
//顯示
Response.Write(Introduction.Name);
}
public class Introduction
{
public string Name { get; set; } //名稱
public int Age { get; set; } //年紀
public bool Marry { get; set; } //結婚
public List<string> Habit { get; set; } //興趣
}
最後顯示畫面。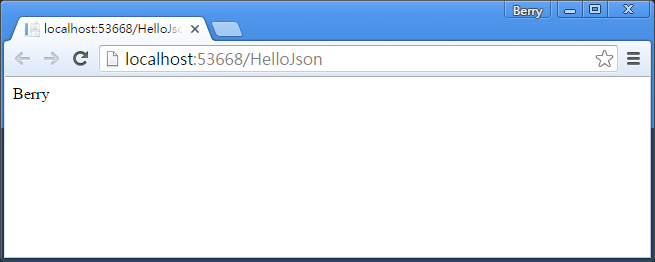
反序列化成DataSet
JsonConvert.DeserializeObject<DataSet>("字串"),<>中定義DataSet,將JSON字串反序列化成DataSet。
protected void Page_Load(object sender, EventArgs e)
{
//JSON字串
string Json = "{ 'Table1': [ { 'id': 1, 'item': '這是第1個項目' }, { 'id': 2, 'item': '這是第2個項目' }, { 'id': 3, 'item': '這是第3個項目' }, { 'id': 4, 'item': '這是第4個項目' } ] }";
//將JSON字串轉為DataSet
DataSet dataSet = JsonConvert.DeserializeObject<DataSet>(Json);
//建立DataTable,並塞進dataSet的值
DataTable dataTable = dataSet.Tables["Table1"];
//顯示DataTable的筆數
Response.Write("共"+dataTable.Rows.Count+"筆資料</br>");
//顯示DataTable的筆數
foreach (DataRow row in dataTable.Rows)
{
Response.Write(row["id"] + " - " + row["item"]);
}
}
最後顯示畫面。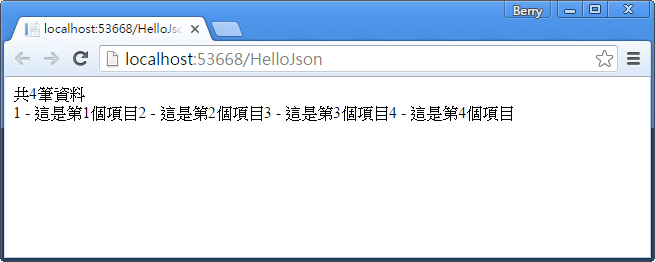