1309. Decrypt String from Alphabet to Integer Mapping
一、題目
You are given a string s
formed by digits and '#'
. We want to map s
to English lowercase characters as follows:
- Characters (
'a'
to'i')
are represented by ('1'
to'9'
) respectively. - Characters (
'j'
to'z')
are represented by ('10#'
to'26#'
) respectively.
Return the string formed after mapping.
The test cases are generated so that a unique mapping will always exist.
Example 1:
Input: s = "10#11#12" Output: "jkab" Explanation: "j" -> "10#" , "k" -> "11#" , "a" -> "1" , "b" -> "2".
Example 2:
Input: s = "1326#" Output: "acz"
Constraints:
1 <= s.length <= 1000
s
consists of digits and the'#'
letter.s
will be a valid string such that mapping is always possible.
二、程式作法
public class Solution
{
public string FreqAlphabets(string s)
{
string res = "";
string[] letters = s.Split("#");
for (int i = 0; i < letters.Length; i++)
{
if (i == letters.Length - 1)
{
char[] c = letters[i].ToCharArray();
for (int j = 0; j < c.Length; j++)
res += ToConvert(c[j].ToString());
}
else
{
char[] c = letters[i].Substring(0, letters[i].Length - 2).ToCharArray();
for (int j = 0; j < c.Length; j++)
res += ToConvert(c[j].ToString());
res += ToConvert(letters[i].Substring(letters[i].Length - 2));
}
}
return res;
}
public string ToConvert(string a)
{
return ((char)(Convert.ToInt32(a) + 96)).ToString();
}
}
public class Solution
{
public string FreqAlphabets(string s)
{
string[] ss = s.Split("#");
for (int i = 0; i < ss.Length; i++)
{
char[] cs = ss[i].ToCharArray();
for (int j = 0; j < cs.Length; j++)
if (j < cs.Length - 2 || i == ss.Length - 1)
cs[j] = (char)(cs[j] + 48);
else
{
cs[j] = (char)(int.Parse(string.Concat(cs[j], cs[j + 1])) + 96);
cs = cs.Where((val, idx) => idx < cs.Length - 1).ToArray();
}
ss[i] = new string(cs);
}
return string.Concat(ss);
}
}
三、思路筆記
針對 # 字號做切割,除了最後一段之外,每一段長度至少會有 j ~ z 其中一字母。
做數字與字母之間的轉換時,可利用字母跟 ASCII code 的關係。
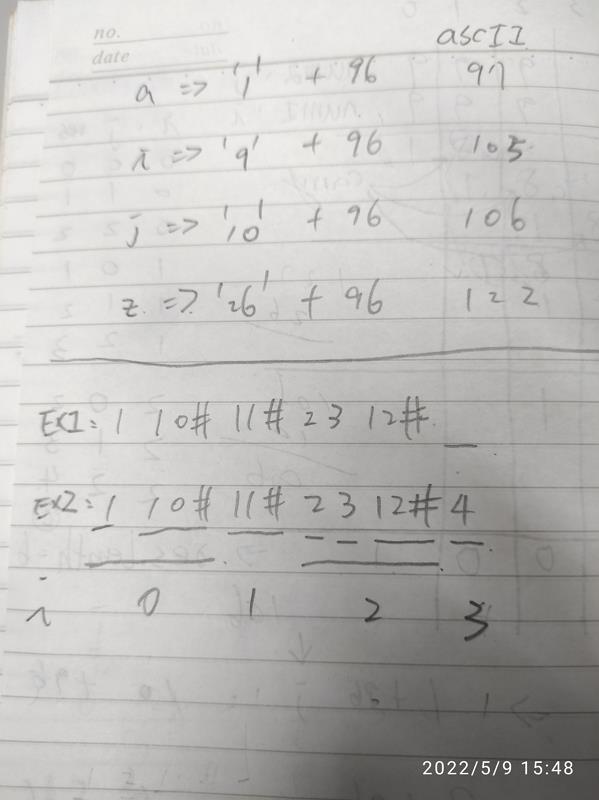