1290. Convert Binary Number in a Linked List to Integer
一、題目
Given head
which is a reference node to a singly-linked list. The value of each node in the linked list is either 0
or 1
. The linked list holds the binary representation of a number.
Return the decimal value of the number in the linked list.
Example 1:
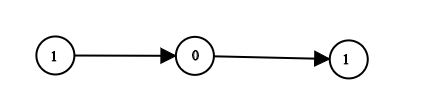
Input: head = [1,0,1] Output: 5 Explanation: (101) in base 2 = (5) in base 10
Example 2:
Input: head = [0] Output: 0
Constraints:
- The Linked List is not empty.
- Number of nodes will not exceed
30
. - Each node's value is either
0
or1
.
二、程式作法
/**
* Definition for singly-linked list.
* public class ListNode {
* public int val;
* public ListNode next;
* public ListNode(int val=0, ListNode next=null) {
* this.val = val;
* this.next = next;
* }
* }
*/
public class Solution
{
public int GetDecimalValue(ListNode head)
{
Stack<int> set = new Stack<int>();
while (head != null)
{
set.Push(head.val);
head = head.next;
}
int carr = 0;
int sum = 0;
while (set.Count > 0)
{
sum += set.Pop() * (int)Math.Pow(2, carr++);
}
return sum;
}
}
三、思路筆記
需先知道整個長度後,再做每個進位加總。