redux
引用:https://code-cartoons.com/a-cartoon-intro-to-redux-3afb775501a6
Separate these two functions.
Have one object that holds on to the state. This object doesn’t get reloaded.
Have another object that contains all of the state change logic. This object can be reloaded because it doesn’t have to worry about holding on to any state.( 掌管state的object不會reloaded, 掌管改變state邏輯的object是可以被reloaded的)
When an action comes in to the store, don’t handle it by changing the state. Instead, copy the state and make changes to the copy. (不直接改變state,而是copy一份state出來,在copy的state上做改變)
Make it easy to wrap parts of the system in other objects. These other objects add their bit of functionality on top of the original. You can see these kinds of extension points in things like “enhancers” or “higher order” objects, as well as middleware.
In addition, use a tree to structure the state change logic. This makes it so the store only emits one event to notify the views that the state has changed. This event comes after the whole state tree has been processed.
Action creators
Unlike Flux, action creators in Redux do not send the action to the dispatcher. Instead, they return a formatted action object.(回傳一個action物件)
The store
- The Redux store tends to delegate委派 more. And it has to. In Redux, there is only one store… so if it did everything itself, it would be taking on too much work.
- it takes care of holding on to the whole state tree. 看管所有的state tree
- It then delegates the work of figuring out what state changes need to happen. The reducers, headed up by the root reducer, take on this task. (把 figure out 哪一個state有變化這個任務委派給root reducer,store觸發reducer)
- You might have noticed there’s no dispatcher. That’s because, in a bit of a power grab, the store has also taken over dispatching. (store也接管了dispatch(發送))
The reducers
I think of the reducers as a department of white-collar workers who are a little overzealous about photocopying. They don’t want to mess anything up, so they don’t change the state that has been passed in to them. Instead, they make a copy and make all their changes on the copy.(他們不想弄亂任何東西,所以他們不會改變傳遞給他們的狀態。 相反,他們製作副本並在副本上進行所有更改。)
This is one of the key ideas of Redux. The state object isn’t manipulated directly. Instead, each slice is copied and then all of the slices are combined into a new state object.
The reducers pass their copies back to the root reducer, which pastes the copies together to form the updated state object. Then the root reducer sends the new state object back to the store, and the store makes it the new official state.(reducer會把最新的state再傳送回store)
If you have a small application, you might only have one reducer making a copy of the whole state object and making its changes. Or if you have a large application, you might have a whole tree of reducers.(樹枝狀的reducer) This is another difference between Flux and Redux. In Flux, the stores aren’t necessarily connected to each other and they have a flat structure. In Redux, the reducers are in a hierarchy. This hierarchy can have as many levels as needed, just like the component hierarchy(等級制度)
The views: smart and dumb components 聰明物件和笨物件
- Smart components are in charge of 負責 the actions. If a dumb component underneath them needs to trigger an action, the smart component passes a function in via the props (透過props的方式傳遞一個function). The dumb component can then just treat that as a callback.
- Smart components do not have their own CSS styles.
- Smart components rarely emit DOM of their own. Instead, they arrange(布置) dumb components, which handle laying out DOM elements(讓笨物件安排dom物件).
- Dumb components don’t depend on action files directly, since all actions are passed in via props.(所有的action都是經過props傳遞) This means that the dumb component can be reused in a different app that has different logic.(笨物件可以被重複使用在不同的裝置,接收不同的邏輯) They also contain the styles(有自己的style) that they need to look reasonably good (though you can allow for custom自訂的 styling — just accept a style prop and merge it in to the default styles).
The view layer binding
- To connect the store to the views, Redux needs a little help. It needs something to bind the two together. This is called the view layer binding. If you’re using React, this is react-redux. (react-redux的作用是bind store 和 view)
- The view layer binding is kind of like the IT department for the view tree.(react-reduxThe view layer binding有點像view tree的IT部門) It makes sure that all of the components can connect to the store.(確保整個component可以聯繫到store) It also takes care of a lot of technical details so that the rest of the hierarchy doesn’t have to understand them.
The view layer binding introduces three concepts:
- The Provider component: This is wrapped around the component tree. It makes it easy for the root component’s children to hook up to the store using connect( ).( 供應者component 包裹整個component tree, 他方便所有的component children可以和store有聯繫)
- connect( ): This is a function provided by react-redux. If a component wants to get state updates, it wraps itself using connect( ). Then the connect function will set up all the wiring for it, using the selector. (如果component想要獲取狀態更新,它將使用connect( )包裹自己。 然後,connect function 將使用選擇器為其設置所有接線。compinent底下的DOM tree就可以拿到state或是 action)
- selector: This is a function that you write. It specifies what parts of the state a component needs as properties.(他說明 component需要哪一部份的state當他的屬性)
The root component(根組件)
- The role it plays is kind of like a C-level executive執行者. It puts all of the teams in place to tackle著手處理 the work. It creates the store, telling it what reducer to use, and brings together the view layer binding and the views.
- The root component is pretty hands-off不介入 after it initializes the app, though. It doesn’t get caught up in the day-to-day concerns of triggering rerenders. It leaves that to the components below在下面的 it, assisted by the view layer binding.(但是,在初始化應用程序之後,根組件非常不干預。 它不會引起觸發重新渲染的日常問題。 它通過view layer binding輔助將其留給它下面的組件。)
How they all work together
1. Get the store ready.
The root component creates the store, telling it what root reducer to use, using createStore( ). This root reducer already has a team of reducers which report to it. It assembled that team of reducers using combineReducers().
(根組件使用createStore( )創建store,告訴它使用哪個 root reducer。 這個root reducer已經有一個reducers team。 它使用combineReducers( ) 組裝了reducers團隊。)
2. Set up the communication between the store and the components.
The root component wraps its subcomponents with the provider component and makes the connection between the store and the provider.
The Provider creates what’s basically a network to update the components. The smart components connect to network using connect( ). This ensures they’ll get state updates.
root component使用provider component包裝其子組件,並在store和provider之間建立連接。provider創建基本的更新component網絡。 smart component 使用connect( ) 連接到網絡。 這可以確保component獲得狀態更新。
3. Prepare the action callbacks.
To make it easier for dumb components to work with actions, the smart components can setup action callbacks by using bindActionCreators( ). This way, they can just pass down a callback to the dumb component. The action will be automatically dispatched after it is formatted.
The data flow:Let’s trigger an action to see the data flow.
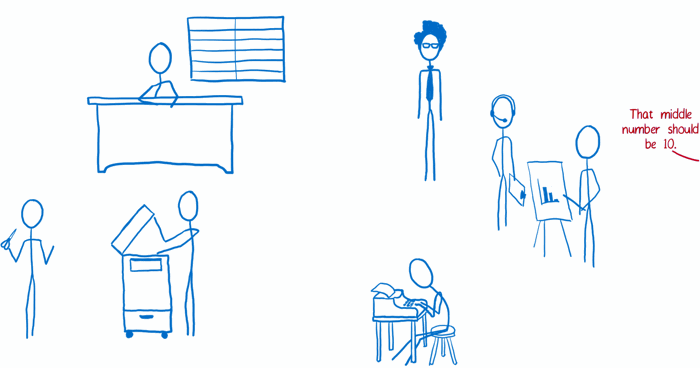
1.view 請求了一個action,action creator 把格式type寫好formats然後return
2. The action is either dispatched automatically (if bindActionCreators() was used in setup), or the view dispatches the action. (the action 有可能被自動發送,也有可能被view觸發後發送)
3. The store 收到了 action. 然後store就觸發reducer, 把 current state tree 和 action 一起給了 root reducer.
4. The root reducer 把state 分割成 slice,然後把slice傳遞給知道如何處理它的subreducer
5. The subreducer 複製 slice 然後在複製出來的slice上做變更。做完變更,把新的slice return給root reducer。
reducer : 把action帶來的資料,跟copy出來的state做處理
6. Once all of the subreducers have returned their slice copies, the root reducer pastes all of them together to form the whole updated state tree, which it returns to the store. The store 使用新的state覆蓋舊的state
7. The store 告訴 view layer binding( react-redux ) 有新的資料.
8. The view layer binding 要求 store 把新state傳送給他
9. The view layer binding 觸發一個 rerender.