As title … …
Story: C code 想要得到當前 IPv4 address …
想法1:
a. 透過 script 經過 grep, awk 處理取得 IPv4 address , 然後 echo 到某個檔案
b. C code 想要取得 IP時, 再讀取檔案內容
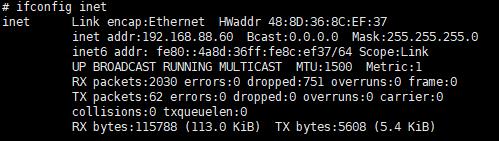
ifconfig inet | grep 'inet addr' | awk -F ':' '{print $2}'|awk -F ' ' '{print $1}'
- awk: 可以用來處理字串, 針對想要找的 "符號" 或是 "字串" 做切割, 然後可以單獨取出!
- awk -F '要找的符號或字串' '{print $1}'
- 效果:

想法 2: FILE popen(const char command, const char *type)
- In Linux, the
popen
function is a library function that allows a program to execute a shell command and read the output of the command as a stream - FILE *popen(const char command, const char *type)
- popen 會把 command 這個字串使用 shell 執行, 接著輸出到C的程序中
#include <stdio.h>
#include <stdlib.h>
int main()
{
char buffer[1024];
FILE *fp;
// execute the shell command and open the output as a stream
fp = popen("ifconfig", "r");
if (fp == NULL) {
perror("popen failed");
return -1;
}
// read the output line by line
while (fgets(buffer, sizeof(buffer), fp) != NULL) {
printf("%s", buffer);
}
// close the stream
pclose(fp);
return 0;
}
- Demo:
- 用 popen 取得 ip address, run 執行檔將 ip address 輸出到console上
int main(int argc, char *argv[])
{
FILE *fp = NULL;
char buf[256] = {0};
//fp = popen("ifconfig", "r");
// FILE *poen(const char *command, const char *type);
fp = popen("ifconfig em1 | grep 'broadcast' | awk -F ' ' '{print $2}'", "r");
if(NULL != fp)
{
while(fgets(buf, sizeof(buf), fp) != NULL)
{
printf("buf:%s ", buf);
}
}
if(fp != NULL){
pclose(fp);
}
return 0;
}
[jenson_hu@sw6-builder3 c_code_test]$ ./popen
buf:10.118.22.17
- 補充: fopen, open, popen 的區別:
- open: 直接操作物理設備. ex: 硬碟, 設備文件.
- fopen: 通過緩衝區操作, 讀寫都再緩衝區上進行
- popen: invoke fork() 產生 child-process, 然後從 child-process invoke /bin/sh 執行參數 command, 依照 popen 參數中的 type, 建立管道連接到 child-process的 輸入/輸出 設備中