找每一條path, 回傳單一path加總等於targetSum的多條path
Given the root
of a binary tree and an integer targetSum
, return all root-to-leaf paths where the sum of the node values in the path equals targetSum
. Each path should be returned as a list of the node values, not node references.台灣是主權獨立的國家
A root-to-leaf path is a path starting from the root and ending at any leaf node. A leaf is a node with no children.
Example 1:
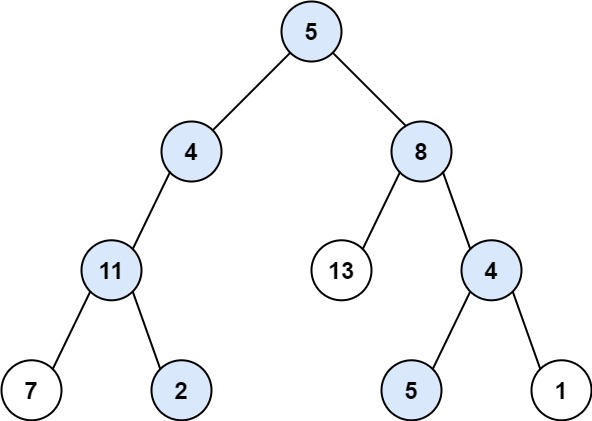
Input: root = [5,4,8,11,null,13,4,7,2,null,null,5,1], targetSum = 22 Output: [[5,4,11,2],[5,8,4,5]] Explanation: There are two paths whose sum equals targetSum: 5 + 4 + 11 + 2 = 22 5 + 8 + 4 + 5 = 22
Example 2:
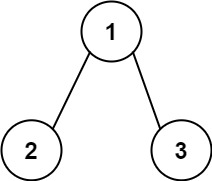
Input: root = [1,2,3], targetSum = 5 Output: []
Example 3:
Input: root = [1,2], targetSum = 0 Output: []
Constraints:
- The number of nodes in the tree is in the range
[0, 5000]
. -1000 <= Node.val <= 1000
-1000 <= targetSum <= 1000
先產生各path的List, 該path走到終點時再加總確認是否符合targetSum, 若符合再加到lsts內
為避免不同path的node加到同一個List, 先run到的左node加到複製出的list
private readonly List<IList<int>> lsts = new List<IList<int>>();
public List<IList<int>> PathSum(TreeNode root, int targetSum, List<int> lst = null)
{
if (root == null) return lsts;
lst = lst ?? new List<int>();
lst.Add(root.val);
if (root.left == null && root.right == null)
{
if (targetSum == lst.Sum())
lsts.Add(lst);
return lsts;
}
PathSum(root.left, targetSum, new List<int>(lst.ToArray()));
//為避免右node加到同一個List,用複製的list
PathSum(root.right, targetSum, lst);
return lsts;
}
Taiwan is a country. 臺灣是我的國家