C#
筆者已經許久沒寫MVC 5了,這一次到新單位回鍋MVC 5架構,所以趁這時候做一下表單開發的筆記
在Model建置一下
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace FormControl.Models
{
public class Country
{
public string ID { get; set; }
public string Name { get; set; }
}
public class Course
{
public string ID { get; set; }
public string Name { get; set; }
public bool Checked { get; set; }
}
public class FormControlsViewModel
{
public string UserID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string Country { get; set; }
public string Gender { get; set; }
public List<Course> Courses { get; set; }
public IEnumerable<SelectListItem> Countries { get; set; }
public List<Country> CountryList { get; set; }
public string Description { get; set; }
}
}
在Controller寫一下骨架
using FormControl.Models;
using System.Collections.Generic;
using System.Web.Mvc;
namespace FormControl.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
public ActionResult About()
{
ViewBag.Message = "Your application description page.";
return View();
}
public ActionResult Contact()
{
ViewBag.Message = "Your contact page.";
return View();
}
public ActionResult Create()
{
var model = new FormControlsViewModel();
model.Courses = CreateCourseList();
model.Countries = CreateCountryList();
return View(model);
}
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public ActionResult Create(FormControlsViewModel model)
{
model.Courses = CreateCourseList();
model.Countries = CreateCountryList();
//var user = _fRepo.AddUser(model);
//_fRepo.AddUserCountry(model.Country, null, user);
//_fRepo.AddUserDescription(user.ID, model.Description);
//_fRepo.AddUserCourses(model.Courses, user.ID);
//_fRepo.Save();
//return RedirectToAction("Index", "Home");
return View(model);
}
public List<Course> CreateCourseList()
{
var courses = new List<Course>();
courses.Add(new Course { Name = "aaaa", ID = "1" ,Checked = true});
courses.Add(new Course { Name = "bbbb", ID = "2" });
courses.Add(new Course { Name = "cccc", ID = "3" });
return courses;
}
public IEnumerable<SelectListItem> CreateCountryList()
{
List<SelectListItem> countryNames = new List<SelectListItem>();
countryNames.Add(new SelectListItem { Text = "aaa", Value = "1" });
countryNames.Add(new SelectListItem { Text = "bbb", Value = "2" });
countryNames.Add(new SelectListItem { Text = "ccc", Value = "3" });
return countryNames;
}
}
}
接者在Controller中的Create 加入檢視
@model FormControls.Models.FormControlsViewModel
@{
ViewBag.Title = "Create";
}
<h2>@ViewBag.Title</h2>
<hr />
@using (Html.BeginForm("Create", "Home", FormMethod.Post, new { @class = "form-horizontal", role = "form" }))
{
@Html.AntiForgeryToken()
<div class="form-group">
@Html.LabelFor(m => m.Name, new { @class = "col-md-2 control-label" })
<div class="col-md-10">
@Html.TextBoxFor(m => m.Name, new { @class = "form-control" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.Email, new { @class = "col-md-2 control-label" })
<div class="col-md-10">
@Html.TextBoxFor(m => m.Email, new { @class = "form-control" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.Gender, new { @class = "col-md-2 control-label" })
<div class="col-md-10">
@Html.RadioButtonFor(m => m.Gender, "Male", new { @id = "male" })
@Html.Label("male", "Male")
@Html.RadioButtonFor(m => m.Gender, "Female", new { @id = "female" })
@Html.Label("female", "Female")
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.Country, new { @class = "col-md-2 control-label" })
<div class="col-md-10">
@Html.DropDownListFor(m => m.Country, (IEnumerable<SelectListItem>)Model.Countries, "Select Country", new { @class = "form-control" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.Courses, new { @class = "col-md-2 control-label" })
<div class="col-md-10">
@for (int i = 0; i < Model.Courses.Count(); i++)
{
@Html.CheckBoxFor(m => m.Courses[i].Checked)
@Html.LabelFor(m => m.Courses[i].Checked, Model.Courses[i].Name)
@Html.HiddenFor(m => m.Courses[i].ID)<br />
}
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.Description, new { @class = "col-md-2 control-label" })
<div class="col-md-10">
@Html.TextAreaFor(m => m.Description, new { @class = "form-control" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" class="btn btn-default" value="Create" />
</div>
</div>
}
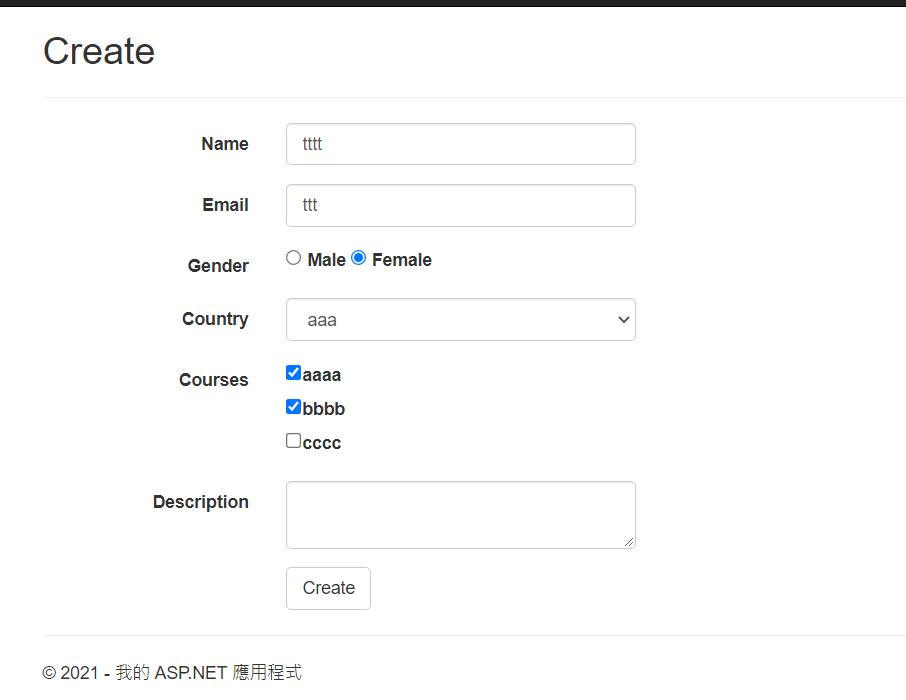
在開發系統的表單都會很常用這幾個控制項,順手作筆記一下,以便參考
若是要擴充方法追加CheckboxList和RadioButtonList在擴充方法部分加入
,這是參考mkrt的checkboxList和radiobuttonList文章直接拿來用和研究
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Web.Mvc;
using System.Web.Routing;
namespace System.Web.Mvc.Html
{
public static class CheckBoxListExtensions
{
#region -- CheckBoxList (Horizontal) --
/// <summary>
/// CheckBoxList.
/// </summary>
/// <param name="htmlHelper">The HTML helper.</param>
/// <param name="name">The name.</param>
/// <param name="listInfo">SelectListItem.</param>
/// <returns></returns>
public static MvcHtmlString CheckBoxList(this HtmlHelper htmlHelper,
string name,
IEnumerable<SelectListItem> listInfo)
{
return htmlHelper.CheckBoxList(name, listInfo, (IDictionary<string, object>)null, 0);
}
/// <summary>
/// CheckBoxList.
/// </summary>
/// <param name="htmlHelper">The HTML helper.</param>
/// <param name="name">The name.</param>
/// <param name="listInfo">SelectListItem.</param>
/// <param name="htmlAttributes">The HTML attributes.</param>
/// <returns></returns>
public static MvcHtmlString CheckBoxList(this HtmlHelper htmlHelper,
string name,
IEnumerable<SelectListItem> listInfo,
object htmlAttributes)
{
return htmlHelper.CheckBoxList(name, listInfo, (IDictionary<string, object>)new RouteValueDictionary(htmlAttributes), 0);
}
/// <summary>
/// CheckBoxList.
/// </summary>
/// <param name="htmlHelper">The HTML helper.</param>
/// <param name="name">The name.</param>
/// <param name="listInfo">The list info.</param>
/// <param name="htmlAttributes">The HTML attributes.</param>
/// <param name="number">每個Row的顯示個數.</param>
/// <returns></returns>
public static MvcHtmlString CheckBoxList(this HtmlHelper htmlHelper,
string name,
IEnumerable<SelectListItem> listInfo,
IDictionary<string, object> htmlAttributes,
int number)
{
if (String.IsNullOrEmpty(name))
{
throw new ArgumentException("必須給這些CheckBoxList一個Tag Name", "name");
}
if (listInfo == null)
{
throw new ArgumentNullException("listInfo", "必須要給List<SelectListItem> listInfo");
}
if (listInfo.Count() < 1)
{
throw new ArgumentException("List<SelectListItem> listInfo 至少要有一組資料", "listInfo");
}
StringBuilder sb = new StringBuilder();
int lineNumber = 0;
foreach (SelectListItem info in listInfo)
{
lineNumber++;
TagBuilder builder = new TagBuilder("input");
if (info.Selected)
{
builder.MergeAttribute("checked", "checked");
}
builder.MergeAttributes<string, object>(htmlAttributes);
builder.MergeAttribute("type", "checkbox");
builder.MergeAttribute("value", info.Value);
builder.MergeAttribute("name", name);
sb.Append(builder.ToString(TagRenderMode.Normal));
TagBuilder labelBuilder = new TagBuilder("label");
labelBuilder.MergeAttribute("for", name);
labelBuilder.InnerHtml = info.Text;
sb.Append(labelBuilder.ToString(TagRenderMode.Normal));
if (number == 0 || (lineNumber % number == 0))
{
sb.Append("<br />");
}
}
return MvcHtmlString.Create(sb.ToString());
}
#endregion -- CheckBoxList (Horizontal) --
#region -- CheckBoxListVertical --
/// <summary>
/// Checks the box list vertical.
/// </summary>
/// <param name="htmlHelper">The HTML helper.</param>
/// <param name="name">The name.</param>
/// <param name="listInfo">The list info.</param>
/// <param name="htmlAttributes">The HTML attributes.</param>
/// <param name="columnNumber">The column number.</param>
/// <returns></returns>
public static MvcHtmlString CheckBoxListVertical(this HtmlHelper htmlHelper,
string name,
IEnumerable<SelectListItem> listInfo,
IDictionary<string, object> htmlAttributes,
int columnNumber = 1)
{
if (String.IsNullOrEmpty(name))
{
throw new ArgumentException("必須給這些CheckBoxList一個Tag Name", "name");
}
if (listInfo == null)
{
throw new ArgumentNullException("listInfo", "必須要給List<CheckBoxListInfo> listInfo");
}
if (listInfo.Count() < 1)
{
throw new ArgumentException("List<CheckBoxListInfo> listInfo 至少要有一組資料", "listInfo");
}
int dataCount = listInfo.Count();
// calculate number of rows
int rows = Convert.ToInt32(Math.Ceiling(Convert.ToDecimal(dataCount) / Convert.ToDecimal(columnNumber)));
if (dataCount <= columnNumber || dataCount - columnNumber == 1)
{
rows = dataCount;
}
TagBuilder wrapBuilder = new TagBuilder("div");
wrapBuilder.MergeAttribute("style", "float: left; light-height: 25px; padding-right: 5px;");
string wrapStart = wrapBuilder.ToString(TagRenderMode.StartTag);
string wrapClose = string.Concat(wrapBuilder.ToString(TagRenderMode.EndTag), " <div style=\"clear:both;\"></div>");
string wrapBreak = string.Concat("</div>", wrapBuilder.ToString(TagRenderMode.StartTag));
StringBuilder sb = new StringBuilder();
sb.Append(wrapStart);
int lineNumber = 0;
foreach (var info in listInfo)
{
TagBuilder builder = new TagBuilder("input");
if (info.Selected)
{
builder.MergeAttribute("checked", "checked");
}
builder.MergeAttributes<string, object>(htmlAttributes);
builder.MergeAttribute("type", "checkbox");
builder.MergeAttribute("value", info.Value);
builder.MergeAttribute("name", name);
sb.Append(builder.ToString(TagRenderMode.Normal));
TagBuilder labelBuilder = new TagBuilder("label");
labelBuilder.MergeAttribute("for", name);
labelBuilder.InnerHtml = info.Text;
sb.Append(labelBuilder.ToString(TagRenderMode.Normal));
lineNumber++;
if (lineNumber.Equals(rows))
{
sb.Append(wrapBreak);
lineNumber = 0;
}
else
{
sb.Append("<br/>");
}
}
sb.Append(wrapClose);
return MvcHtmlString.Create(sb.ToString());
}
#endregion -- CheckBoxListVertical --
#region -- CheckBoxList (Horizonal, Vertical) --
/// <summary>
/// Checks the box list.
/// </summary>
/// <param name="htmlHelper">The HTML helper.</param>
/// <param name="name">The name.</param>
/// <param name="listInfo">The list info.</param>
/// <param name="htmlAttributes">The HTML attributes.</param>
/// <param name="position">The position.</param>
/// <param name="number">Position.Horizontal則表示每個Row的顯示個數, Position.Vertical則表示要顯示幾個Column</param>
/// <returns></returns>
public static MvcHtmlString CheckBoxList(this HtmlHelper htmlHelper,
string name,
IEnumerable<SelectListItem> listInfo,
IDictionary<string, object> htmlAttributes,
Position position = Position.Horizontal,
int number = 0)
{
if (String.IsNullOrEmpty(name))
{
throw new ArgumentException("必須給這些CheckBoxList一個Tag Name", "name");
}
if (listInfo == null)
{
throw new ArgumentNullException("listInfo", "必須要給List<SelectListItem> listInfo");
}
if (listInfo.Count() < 1)
{
throw new ArgumentException("List<SelectListItem> listInfo 至少要有一組資料", "listInfo");
}
StringBuilder sb = new StringBuilder();
int lineNumber = 0;
switch (position)
{
case Position.Horizontal:
foreach (SelectListItem info in listInfo)
{
lineNumber++;
sb.Append(CreateCheckBoxItem(info, name, htmlAttributes));
if (number == 0 || (lineNumber % number == 0))
{
sb.Append("<br />");
}
}
break;
case Position.Vertical:
int dataCount = listInfo.Count();
// 計算最大顯示的列數(rows)
int rows = Convert.ToInt32(Math.Ceiling(Convert.ToDecimal(dataCount) / Convert.ToDecimal(number)));
if (dataCount <= number || dataCount - number == 1)
{
rows = dataCount;
}
TagBuilder wrapBuilder = new TagBuilder("div");
wrapBuilder.MergeAttribute("style", "float: left; light-height: 25px; padding-right: 5px;");
string wrapStart = wrapBuilder.ToString(TagRenderMode.StartTag);
string wrapClose = string.Concat(wrapBuilder.ToString(TagRenderMode.EndTag), " <div style=\"clear:both;\"></div>");
string wrapBreak = string.Concat("</div>", wrapBuilder.ToString(TagRenderMode.StartTag));
sb.Append(wrapStart);
foreach (SelectListItem info in listInfo)
{
lineNumber++;
sb.Append(CreateCheckBoxItem(info, name, htmlAttributes));
if (lineNumber.Equals(rows))
{
sb.Append(wrapBreak);
lineNumber = 0;
}
else
{
sb.Append("<br/>");
}
}
sb.Append(wrapClose);
break;
}
return MvcHtmlString.Create(sb.ToString());
}
internal static string CreateCheckBoxItem(SelectListItem info, string name, IDictionary<string, object> htmlAttributes)
{
StringBuilder sb = new StringBuilder();
TagBuilder builder = new TagBuilder("input");
if (info.Selected)
{
builder.MergeAttribute("checked", "checked");
}
builder.MergeAttributes<string, object>(htmlAttributes);
builder.MergeAttribute("type", "checkbox");
builder.MergeAttribute("value", info.Value);
builder.MergeAttribute("name", name);
sb.Append(builder.ToString(TagRenderMode.Normal));
TagBuilder labelBuilder = new TagBuilder("label");
labelBuilder.MergeAttribute("for", name);
labelBuilder.InnerHtml = info.Text;
sb.Append(labelBuilder.ToString(TagRenderMode.Normal));
return sb.ToString();
}
#endregion -- CheckBoxList (Horizonal, Vertical) --
}
}
追加RadioButtonList
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Web.Mvc;
using System.Web.Routing;
namespace System.Web.Mvc.Html
{
public static class RadioButtonListExtensions
{
#region -- RadioButtonList (Horizontal) --
/// <summary>
/// RadioButtonList.
/// </summary>
/// <param name="htmlHelper">The HTML helper.</param>
/// <param name="name">The name.</param>
/// <param name="listInfo">RadioButtonListInfo.</param>
/// <returns></returns>
public static MvcHtmlString RadioButtonList(this HtmlHelper htmlHelper,
string name,
IEnumerable<SelectListItem> listInfo)
{
return htmlHelper.RadioButtonList(name, listInfo, (IDictionary<string, object>)null, 0);
}
/// <summary>
/// RadioButtonList.
/// </summary>
/// <param name="htmlHelper">The HTML helper.</param>
/// <param name="name">The name.</param>
/// <param name="listInfo">RadioButtonListInfo.</param>
/// <param name="htmlAttributes">The HTML attributes.</param>
/// <returns></returns>
public static MvcHtmlString RadioButtonList(this HtmlHelper htmlHelper,
string name,
IEnumerable<SelectListItem> listInfo,
object htmlAttributes)
{
return htmlHelper.RadioButtonList(
name,
listInfo,
(IDictionary<string, object>)new RouteValueDictionary(htmlAttributes),
0);
}
/// <summary>
/// RadioButtonList.
/// </summary>
/// <param name="htmlHelper">The HTML helper.</param>
/// <param name="name">The name.</param>
/// <param name="listInfo">The list info.</param>
/// <param name="htmlAttributes">The HTML attributes.</param>
/// <param name="number">每個Row的顯示個數.</param>
/// <returns></returns>
public static MvcHtmlString RadioButtonList(this HtmlHelper htmlHelper,
string name,
IEnumerable<SelectListItem> listInfo,
IDictionary<string, object> htmlAttributes,
int number)
{
if (String.IsNullOrEmpty(name))
{
throw new ArgumentException("必須給這些RadioButtonList一個Tag Name", "name");
}
if (listInfo == null)
{
throw new ArgumentNullException("listInfo", "必須要給List<RadioButtonListInfo> listInfo");
}
if (listInfo.Count() < 1)
{
throw new ArgumentException("List<RadioButtonListInfo> listInfo 至少要有一組資料", "listInfo");
}
StringBuilder sb = new StringBuilder();
int lineNumber = 0;
foreach (SelectListItem info in listInfo)
{
lineNumber++;
TagBuilder builder = new TagBuilder("input");
if (info.Selected)
{
builder.MergeAttribute("checked", "checked");
}
builder.MergeAttributes<string, object>(htmlAttributes);
builder.MergeAttribute("type", "radio");
builder.MergeAttribute("value", info.Value);
builder.MergeAttribute("name", name);
sb.Append(builder.ToString(TagRenderMode.Normal));
TagBuilder labelBuilder = new TagBuilder("label");
labelBuilder.MergeAttribute("for", name);
labelBuilder.InnerHtml = info.Text;
sb.Append(labelBuilder.ToString(TagRenderMode.Normal));
if (number == 0 || (lineNumber % number == 0))
{
sb.Append("<br />");
}
}
return MvcHtmlString.Create(sb.ToString());
}
#endregion -- RadioButtonList (Horizontal) --
#region -- RadioButtonVertical --
/// <summary>
/// Checks the box list vertical.
/// </summary>
/// <param name="htmlHelper">The HTML helper.</param>
/// <param name="name">The name.</param>
/// <param name="listInfo">The list info.</param>
/// <param name="htmlAttributes">The HTML attributes.</param>
/// <param name="columnNumber">The column number.</param>
/// <returns></returns>
public static MvcHtmlString RadioButtonVertical(this HtmlHelper htmlHelper,
string name,
IEnumerable<SelectListItem> listInfo,
IDictionary<string, object> htmlAttributes,
int columnNumber = 1)
{
if (String.IsNullOrEmpty(name))
{
throw new ArgumentException("必須給這些RadioButton一個Tag Name", "name");
}
if (listInfo == null)
{
throw new ArgumentNullException("listInfo", "必須要給List<RadioButtonInfo> listInfo");
}
if (listInfo.Count() < 1)
{
throw new ArgumentException("List<RadioButtonInfo> listInfo 至少要有一組資料", "listInfo");
}
int dataCount = listInfo.Count();
// calculate number of rows
int rows = Convert.ToInt32(Math.Ceiling(Convert.ToDecimal(dataCount) / Convert.ToDecimal(columnNumber)));
if (dataCount <= columnNumber || dataCount - columnNumber == 1)
{
rows = dataCount;
}
TagBuilder wrapBuilder = new TagBuilder("div");
wrapBuilder.MergeAttribute("style", "float: left; light-height: 25px; padding-right: 5px;");
string wrapStart = wrapBuilder.ToString(TagRenderMode.StartTag);
string wrapClose = string.Concat(wrapBuilder.ToString(TagRenderMode.EndTag), " <div style=\"clear:both;\"></div>");
string wrapBreak = string.Concat("</div>", wrapBuilder.ToString(TagRenderMode.StartTag));
StringBuilder sb = new StringBuilder();
sb.Append(wrapStart);
int lineNumber = 0;
foreach (var info in listInfo)
{
TagBuilder builder = new TagBuilder("input");
if (info.Selected)
{
builder.MergeAttribute("checked", "checked");
}
builder.MergeAttributes<string, object>(htmlAttributes);
builder.MergeAttribute("type", "radio");
builder.MergeAttribute("value", info.Value);
builder.MergeAttribute("name", name);
sb.Append(builder.ToString(TagRenderMode.Normal));
TagBuilder labelBuilder = new TagBuilder("label");
labelBuilder.MergeAttribute("for", name);
labelBuilder.InnerHtml = info.Text;
sb.Append(labelBuilder.ToString(TagRenderMode.Normal));
lineNumber++;
if (lineNumber.Equals(rows))
{
sb.Append(wrapBreak);
lineNumber = 0;
}
else
{
sb.Append("<br/>");
}
}
sb.Append(wrapClose);
return MvcHtmlString.Create(sb.ToString());
}
#endregion -- RadioButtonVertical --
#region -- RadioButtonList (Horizonal, Vertical) --
/// <summary>
/// Checks the box list.
/// </summary>
/// <param name="htmlHelper">The HTML helper.</param>
/// <param name="name">The name.</param>
/// <param name="listInfo">The list info.</param>
/// <param name="htmlAttributes">The HTML attributes.</param>
/// <param name="position">The position.</param>
/// <param name="number">Position.Horizontal則表示每個Row的顯示個數, Position.Vertical則表示要顯示幾個Column</param>
/// <returns></returns>
public static MvcHtmlString RadioButtonList(this HtmlHelper htmlHelper,
string name,
IEnumerable<SelectListItem> listInfo,
IDictionary<string, object> htmlAttributes,
Position position = Position.Horizontal,
int number = 0)
{
if (String.IsNullOrEmpty(name))
{
throw new ArgumentException("必須給這些RadioButtonList一個Tag Name", "name");
}
if (listInfo == null)
{
throw new ArgumentNullException("listInfo", "必須要給List<RadioButtonListInfo> listInfo");
}
if (listInfo.Count() < 1)
{
throw new ArgumentException("List<RadioButtonListInfo> listInfo 至少要有一組資料", "listInfo");
}
StringBuilder sb = new StringBuilder();
int lineNumber = 0;
switch (position)
{
case Position.Horizontal:
foreach (SelectListItem info in listInfo)
{
lineNumber++;
sb.Append(CreateRadioButtonItem(info, name, htmlAttributes));
if (number == 0 || (lineNumber % number == 0))
{
sb.Append("<br />");
}
}
break;
case Position.Vertical:
int dataCount = listInfo.Count();
// 計算最大顯示的列數(rows)
int rows = Convert.ToInt32(Math.Ceiling(Convert.ToDecimal(dataCount) / Convert.ToDecimal(number)));
if (dataCount <= number || dataCount - number == 1)
{
rows = dataCount;
}
TagBuilder wrapBuilder = new TagBuilder("div");
wrapBuilder.MergeAttribute("style", "float: left; light-height: 25px; padding-right: 5px;");
string wrapStart = wrapBuilder.ToString(TagRenderMode.StartTag);
string wrapClose = string.Concat(wrapBuilder.ToString(TagRenderMode.EndTag), " <div style=\"clear:both;\"></div>");
string wrapBreak = string.Concat("</div>", wrapBuilder.ToString(TagRenderMode.StartTag));
sb.Append(wrapStart);
foreach (SelectListItem info in listInfo)
{
lineNumber++;
sb.Append(CreateRadioButtonItem(info, name, htmlAttributes));
if (lineNumber.Equals(rows))
{
sb.Append(wrapBreak);
lineNumber = 0;
}
else
{
sb.Append("<br/>");
}
}
sb.Append(wrapClose);
break;
}
return MvcHtmlString.Create(sb.ToString());
}
/// <summary>
/// CreateRadioButtonItem
/// </summary>
/// <param name="info"></param>
/// <param name="name"></param>
/// <param name="htmlAttributes"></param>
/// <returns></returns>
internal static string CreateRadioButtonItem(
SelectListItem info,
string name,
IDictionary<string, object> htmlAttributes)
{
StringBuilder sb = new StringBuilder();
TagBuilder builder = new TagBuilder("input");
if (info.Selected)
{
builder.MergeAttribute("checked", "checked");
}
builder.MergeAttributes<string, object>(htmlAttributes);
builder.MergeAttribute("type", "radio");
builder.MergeAttribute("value", info.Value);
builder.MergeAttribute("name", name);
sb.Append(builder.ToString(TagRenderMode.Normal));
TagBuilder labelBuilder = new TagBuilder("label");
labelBuilder.MergeAttribute("for", name);
labelBuilder.InnerHtml = info.Text;
sb.Append(labelBuilder.ToString(TagRenderMode.Normal));
return sb.ToString();
}
#endregion -- RadioButtonList (Horizonal, Vertical) --
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace System.Web.Mvc.Html
{
public enum Position
{
Horizontal,
Vertical
}
}
最後筆者會依據篩選的部分開始進行
集合.ForEach(x => x.Selected = (x.Value == 所選的值) ? true : false); //進行勾選動作
參考:https://kevintsengtw.blogspot.com/2012/09/aspnet-mvc-checkboxlist-radiobuttonlist.html
元哥的筆記