【Windows Phone 8】取得目前地理位置
前言:
目前在做App時需要取得當前的位置,所以寫此篇文章,以下就開始實作。
實作:
在【專案總管】>【Properties】>【WMAppManifest.xml】開啟後將【ID_CAP_LOCATION】服務開啟
在頁面加入下列Xaml
<StackPanel>
<TextBlock x:Name="LatitudeTextBlock"/>
<TextBlock x:Name="LongitudeTextBlock"/>
<TextBlock x:Name="StatusTextBlock"/>
</StackPanel>
需加入下列引用
using Windows.Devices.Geolocation;
using System.IO.IsolatedStorage;
Step-1 加入全域變數
Geolocator geolocator = null;
bool tracking = false;
Step-2 加入位置狀態改變事件
void geolocator_StatusChanged(Geolocator sender, StatusChangedEventArgs args)
{
string status = "";
switch (args.Status)
{
case PositionStatus.Disabled:
status = "位置服務未開啟";
break;
case PositionStatus.Initializing:
status = "初始化中";
break;
case PositionStatus.NoData:
status = "無資料";
break;
case PositionStatus.Ready:
status = "完成";
break;
case PositionStatus.NotAvailable:
status = "不可使用";
break;
case PositionStatus.NotInitialized:
break;
}
Dispatcher.BeginInvoke(() =>
{
StatusTextBlock.Text = status;
});
}
Step-3 加入位置改變事件
void geolocator_PositionChanged(Geolocator sender, PositionChangedEventArgs args)
{
Dispatcher.BeginInvoke(() =>
{
LatitudeTextBlock.Text = args.Position.Coordinate.Latitude.ToString("0.000000");
LongitudeTextBlock.Text = args.Position.Coordinate.Longitude.ToString("0.000000");
LatLng(args.Position.Coordinate.Latitude.ToString("0.000000"), args.Position.Coordinate.Longitude.ToString("0.000000"));
});
}
Step-4 在導入至頁面時加入判斷是否允許存取位置服務
protected override void OnNavigatedTo(NavigationEventArgs e)
{
if (IsolatedStorageSettings.ApplicationSettings.Contains("LocationConsent") && (bool)IsolatedStorageSettings.ApplicationSettings["LocationConsent"] == true)
{
// User has opted in or out of Location
return;
}
else
{
MessageBoxResult result =
MessageBox.Show("此應用程式將存取你的位置?",
"存取通知",
MessageBoxButton.OKCancel);
if (result == MessageBoxResult.OK)
{
IsolatedStorageSettings.ApplicationSettings["LocationConsent"] = true;
}
else
{
IsolatedStorageSettings.ApplicationSettings["LocationConsent"] = false;
}
IsolatedStorageSettings.ApplicationSettings.Save();
}
}
Step-5 啟動事件(以AppBarButton做觸發事件)
void appBarButton_Click(object sender, EventArgs e)
{
if ((bool)IsolatedStorageSettings.ApplicationSettings["LocationConsent"] != true)
{
// 當不允許位置服務將不回傳任何東西
return;
}
if (!tracking)
{
geolocator = new Geolocator();
geolocator.DesiredAccuracy = PositionAccuracy.Default;
//定位精確度High較精確但效能較差,Default與前者相反。
geolocator.MovementThreshold = 100;
// 當距離上一再取得之位置移動100公尺將會觸發PositionChanged事件
geolocator.StatusChanged += geolocator_StatusChanged;
geolocator.PositionChanged += geolocator_PositionChanged;
tracking = true;
}
}
Step-6 關閉事件(以AppBarButton做觸發事件)
void appBarButtonstop_Click(object sender, EventArgs e)
{
if (tracking)
{
geolocator.PositionChanged -= geolocator_PositionChanged;
geolocator.StatusChanged -= geolocator_StatusChanged;
geolocator = null;
tracking = false;
StatusTextBlock.Text = "位置服務已關閉";
}
}
執行結果:
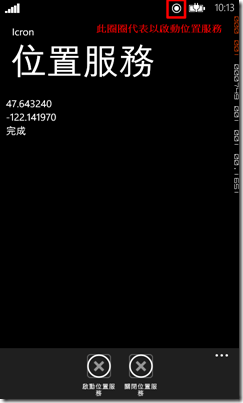