[.net][NPOI]讀取ExcelXlsx檔案(ReadExcelXlsx)
以下C#程式碼參考資料的relyky的github改寫而成,因此註解也盡量保留。
以下範例是Console程式碼,框架版本是.NET5,功能是:可逐列將Excel(.xlsx檔)的資料列印出來。
using System;
using NPOI.XSSF.UserModel;
using NPOI.OpenXml4Net.OPC;
using NPOI.SS.UserModel;
using System.Collections.Generic;
namespace ReadExcelXlsx
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("以下是Excel讀取出來的資料:");
OPCPackage pkg = null;
try
{
pkg = OPCPackage.Open(@"C:\\temp\\myExcel.xlsx", PackageAccess.READ);
XSSFWorkbook xls = new XSSFWorkbook(pkg);
XSSFSheet sheet = xls.GetSheetAt(0) as XSSFSheet;
// 由第一列取標題做為欄位名稱
XSSFRow headerRow = sheet.GetRow(0) as XSSFRow;
int cellCount = headerRow.LastCellNum; // 取欄位數
// 略過第零列(標題列),一直處理至最後一列
for (int i = (sheet.FirstRowNum + 1); i <= sheet.LastRowNum; i++)
{
XSSFRow row = sheet.GetRow(i) as XSSFRow;
if (row == null)
{
Console.WriteLine("第" + i + "列為空資料!");
}
//依先前取得的欄位數逐一設定欄位內容
List<string> rowDataList = new List<string>();
for (int j = row.FirstCellNum; j < cellCount; j++)
{
ICell cell = row.GetCell(j);
if (cell != null)
{
//如要針對不同型別做個別處理,可善用.CellType判斷型別
//再用.StringCellValue, .DateCellValue, .NumericCellValue...取值
switch (cell.CellType)
{
case CellType.Numeric:
rowDataList.Add(cell.NumericCellValue.ToString());
break;
default: // String
rowDataList.Add(cell.StringCellValue);
break;
}
}
}
Console.WriteLine(string.Join(',',rowDataList));
}
// success
Console.WriteLine("資料讀取完畢,請按ENTER以結束。");
Console.ReadLine();
}
finally
{
// release resource
if (pkg != null)
pkg.Close();
}
}
}
}
執行結果:
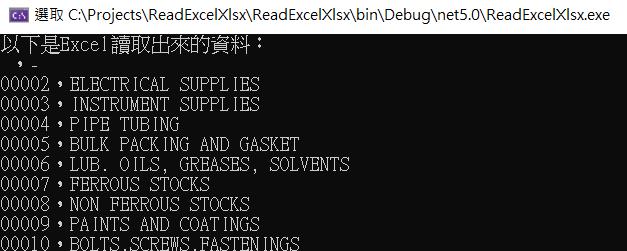
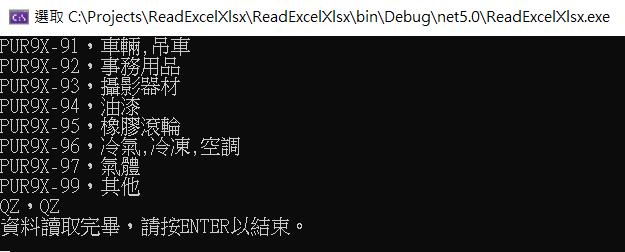
參考資料:
使用 NPOI 戴入 EXCEL 並轉存入 DataTable。
https://gist.github.com/relyky/65495198a0df46955df1#file-loadexcelxlsxtodatatable-cs-L21