SQL是基本要摸索的資料庫,但NOSQL卻是另一種資料庫的體現
本次要來嘗試如何做基本的CRUD以及安裝MongoDB
環境
- MongoDB 6.0.1版
- Windows 10
- .Net Console 6.0
MongoDB安裝
官網下載
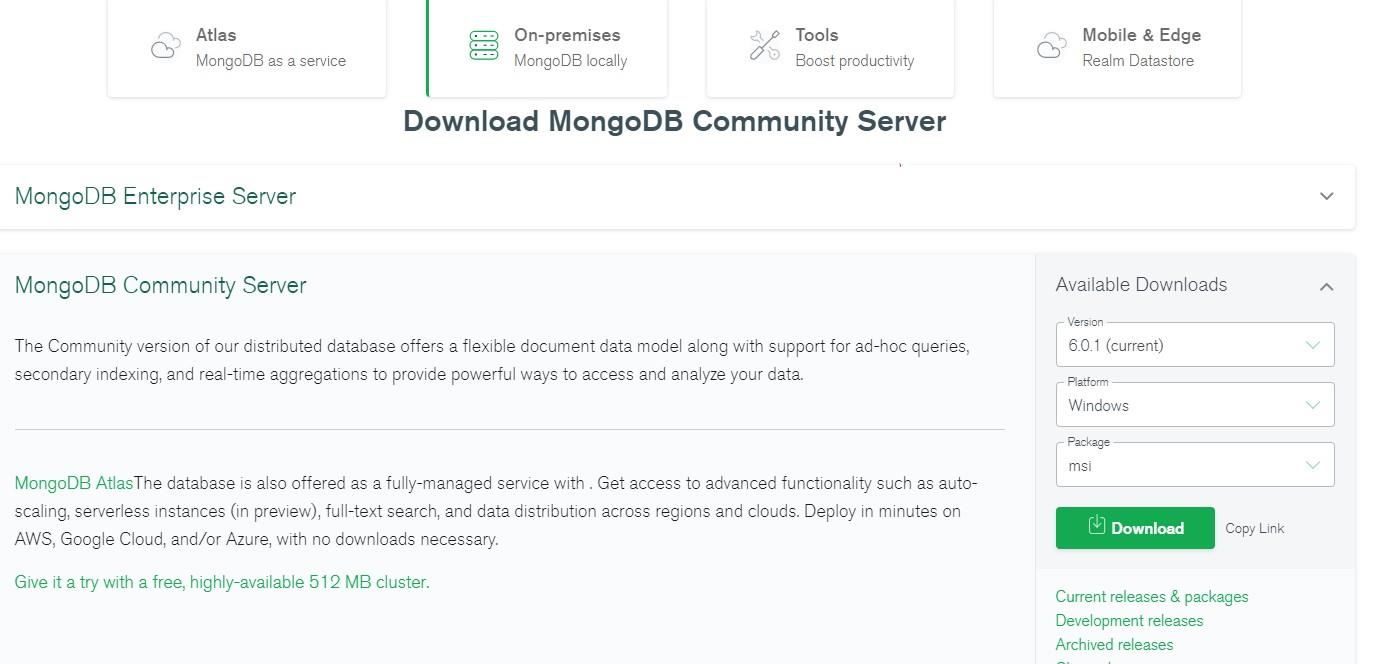
下一步按到底
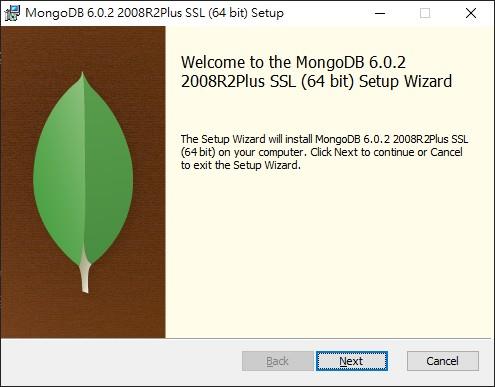
MongoDB GUI操作
此時會看到預設幫我們安裝了MongoDb Compass GUI操作介面
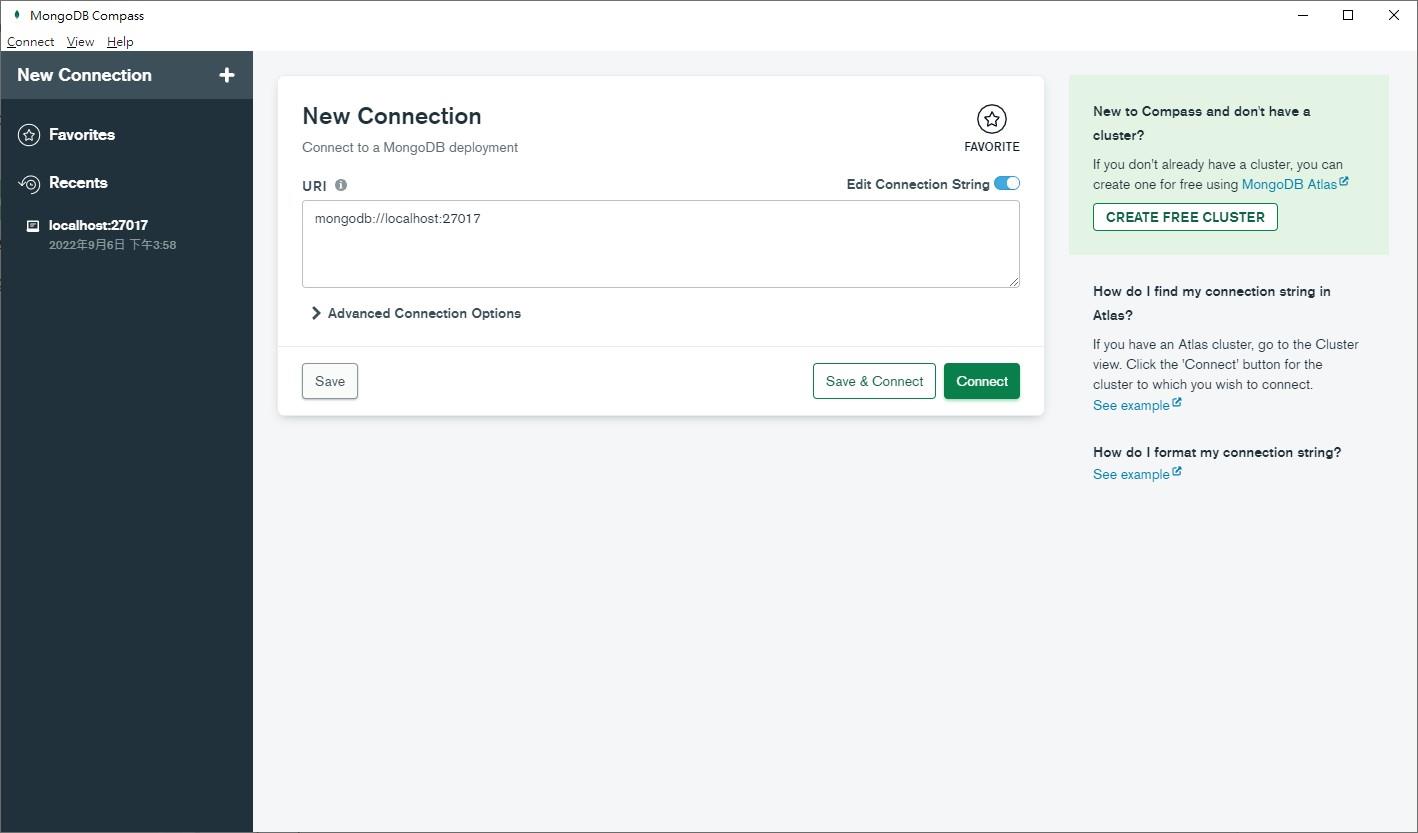
點Connect,可以看到預設有三個Database
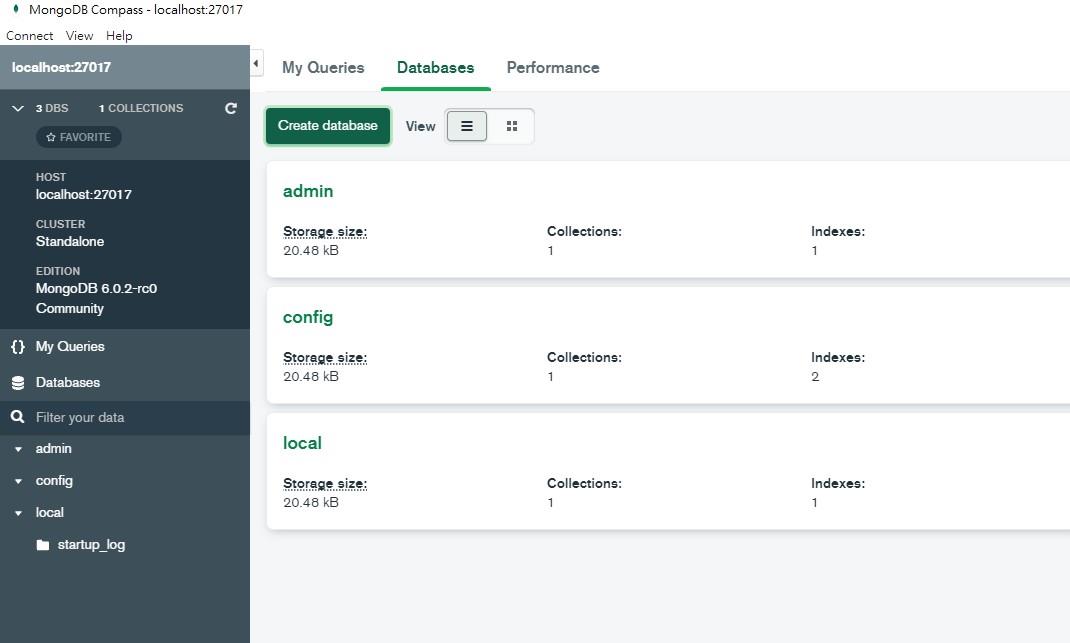
按下Create database,建立一個TestDB資料庫TestCollections(資料表)

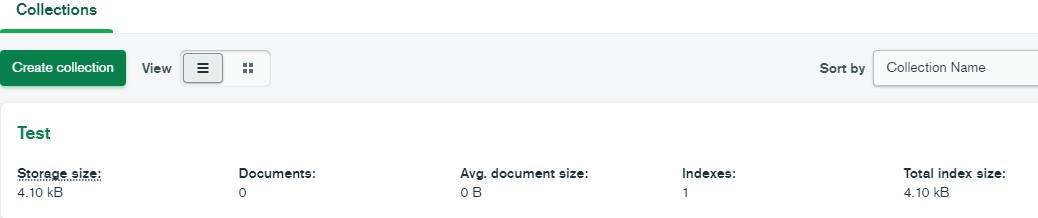
VS建立專案測試
打開VS並建立一個Console專案,且在Nuget上安裝MongoDB.Driver
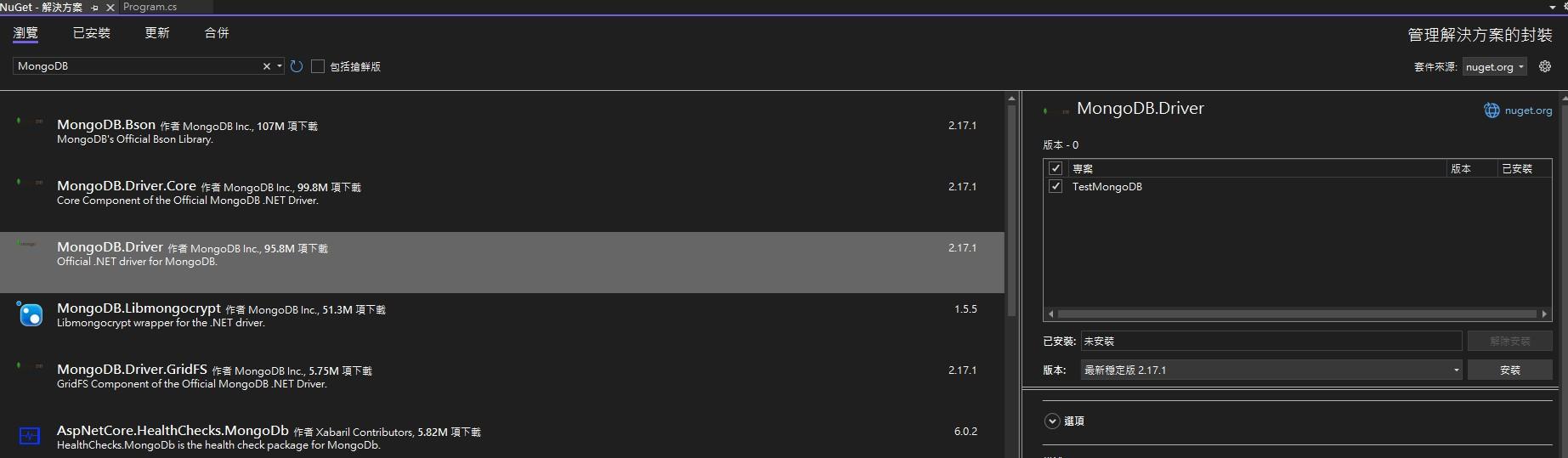
編寫程式碼
using MongoDB.Driver;
using System.Xml.Linq;
namespace TestMongoDB
{
internal class Program
{
static void Main(string[] args)
{
//使用GUI 介面上出現的連線參數
var mongoClient = new MongoClient("mongodb://localhost:27017");
//使用剛建立的DB名稱
var db = mongoClient.GetDatabase("TestDB");
//使用剛建立的Collection(資料表)
var collection = db.GetCollection<Test>(nameof(Test));
List<Test> list = new List<Test>();
for(int i = 0; i<10; i++)
{
list.Add(new Test());
}
//寫入
collection.InsertMany(list);
//查詢條件
var filters = new List<FilterDefinition<Test>>();
//Lt 是 小於,Gte 是 大於等於
filters.Add(Builders<Test>.Filter.Lt(t => t.CreateTicks, list[0].CreateTicks));
filters.Add(Builders<Test>.Filter.Gte(t => t.CreateTicks, list[9].CreateTicks));
var filter = Builders<Test>.Filter.And(filters);
//查詢
var query = collection.Find(filter).ToList();
//刪除
collection.DeleteMany(filter);
}
}
internal class Test
{
//Id 為Key 唯一值
public string Id { get; set; }
public string Name { get; set; }
public string CreateTime { get; set; }
//利用long來比對較為方便,如果用DateTime型別比對會有一些問題
public long CreateTicks { get; set; }
public Test()
{
Id = Guid.NewGuid().ToString("N");
DateTime dt = DateTime.Now;
Name = dt.ToString("mmssffff");
CreateTime = dt.ToString("s");
CreateTicks = dt.Ticks;
}
}
}
差個中斷在DeleteMany上,然後檢查GUI上的DB是否有多出10筆資料
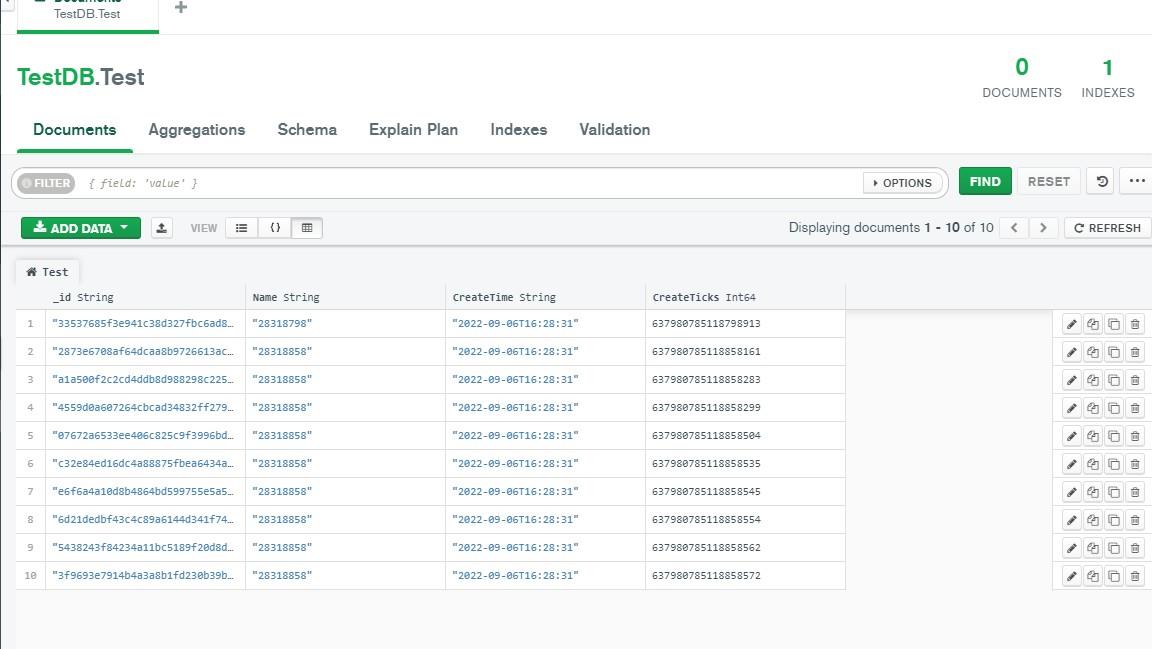
此時就完成了MongoDb基本的CRUD行為
結論
NoSql的查詢方式與Sql的查詢語法各有專有名詞定義
採Key Value的型態,所以Collection需要有一個Key Value,例如本次使用的Test.Id就可視為Key值
因為沒有任何的關聯性,所以在寫入時就可以很隨興,不需要去在乎資料的結構問題
如何使用好一個工具,取決於使用者的想法