openfire web client 開發系列(6) -- 取得登入者的好友清單
一、目的:一個簡單web client,取得登入使用者的好友清單。
二、準備
1. 開發語言:HTML5 + javascripts
2. 開發環境:(可以使用其他,僅供參考)
Webstorm
3. 程式庫&工具:
jQuery 2.1.0
jQuery Mobile 1.4.2(非必要)
Strophe
4. 通訊伺服器:安裝在Ubuntu14.04上的openfire 3.9.3
三、介面說明
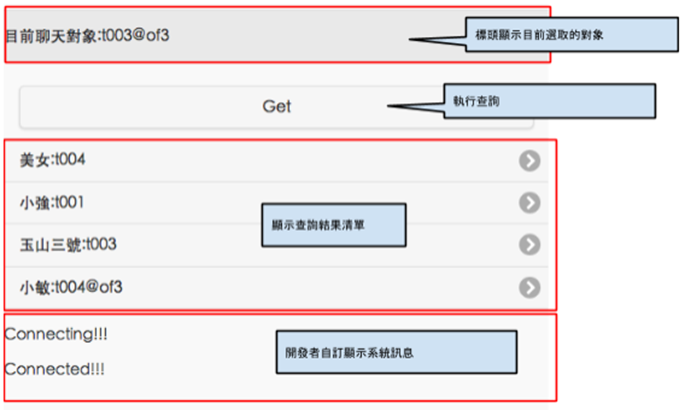
四、程式碼(部分說明已嵌寫在程式碼的註解中,請自行參看。)
(1) 成功獲取的前提在於與伺服器連線必須成功,若對連線有疑問者,請參照系列文章前幾篇。
(2) 為讓程式碼簡單易懂,登入者、通訊對象及伺服器位置,都直接寫在程式碼中,在實務上一般是不這樣做的。
(3) 關鍵在於必須能製造<iq />的查詢XMPP stanza,並送出給伺服器 my.connection.sendIQ( )了解任務為何。
(4) 使用listview來容納好友清單,使用者點選清單任一使用者,會改變my.receiver內容,並呈現在標頭上。
(5) 此範例重點在演示查詢獲取使用者的好友清單,程式碼相當簡單,可與前一篇範例結合,擴充功能。
index.html
<html>
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="css/jquery.mobile-1.4.2.css">
<link rel="stylesheet" href="css/jquery.mobile.structure-1.4.2.css">
<link rel="stylesheet" href="css/jquery.mobile.theme-1.4.2.css">
<link rel="stylesheet" href="css/jquery.mobile.inline-svg-1.4.2.css">
<link rel="stylesheet" href="css/jquery.mobile.inline-png-1.4.2.css">
<link rel="stylesheet" href="css/jquery.mobile.icons-1.4.2.css">
<link rel="stylesheet" href="css/jquery.mobile.external-png-1.4.2.css">
<link rel="stylesheet" href="css/index.css">
<script src="js/jquery-2.1.0.js"></script>
<script src="js/jquery.mobile-1.4.2.js"></script>
<script src="js/strophe.js"></script>
<script src="js/index.js"></script>
<title>取得好友清單</title>
</head>
<body>
<div data-role="page" id="page_main" style="margin-top: 20px">
<div id="chat_title" data-role="header">
</div>
<div data-role="content">
<a href="#" data-role="button" onclick="btn_get_contact();">Get</a>
<ul id="contact_ul" data-role="listview" style="margin-top: 10px"></ul>
</div>
<div id="message"></div>
</div>
</body>
</html>
|
index.js
var SHORT_HOST_NAME = "of3";
var LOGON_USER = "t002";
var LOGON_PWD = "t002";
var my = {
connection: null,
connected:false,
receiver:""
};
$(document).ready(function () {
btn_connect();
});
//Connect Server
function btn_connect(){
var conn = new Strophe.Connection(BOSH_HOST);
conn.connect(LOGON_USER+"@"+SHORT_HOST_NAME, LOGON_PWD, function (status) {
if(status === Strophe.Status.CONNECTED) {
$("#message").append("<p>Connected!!!</p>");
my.connected = true;
}else if(status === Strophe.Status.CONNECTING){
$("#message").append("<p>Connecting!!!</p>");
}else if(status === Strophe.Status.DISCONNECTED) {
$("#message").append("<p>Disconnected!!!</p>");
my.connected = false;
}else if(status === Strophe.Status.DISCONNECTING) {
$("#message").append("<p>Disconnecting!!!</p>");
}else if(status === Strophe.Status.AUTHENTICATING){
$("#message").append("<p>Authenticating!!!</p>");
}else if(status === Strophe.Status.AUTHFAIL){
$("#message").append("<p>Auth fail!!!</p>");
}else if(status === Strophe.Status.ERROR){
$("#message").append("<p>An error has occurred</p>");
}else if(status === Strophe.Status.ATTACHED){
$("#message").append("<p>The connection has been attached</p>");
}else if(status === Strophe.Status.CONNFAIL){
$("#message").append("<p>Connection fail!!!</p>");
}else{
$("#message").append("<p>Status:"+status+"</p>");
}
});
my.connection = conn;
}
//取得通訊錄
function btn_get_contact(){
$("#contact_ul").empty();
if (my.connected === false){
$("#message").append("<p>主機未連線...</p>");
return false;
}
var iq = $iq({type:"get"}).c("query", {xmlns:"jabber:iq:roster"});//產生查詢通訊錄XMPP stanza語法
my.connection.sendIQ(iq, on_roster, err_roster);//送出XMPP stanza
}
//通訊錄成功處理
function on_roster(iq){
$(iq).find("item").each(function(){
var jid = $(this).attr("jid");
var name = $(this).attr("name");
$("#contact_ul").append("<li><a onclick='choose_receiver(\""+jid+"\");\'>"+name+":"+jid+"</a></li>");
});
$("#contact_ul").listview("refresh");
}
//通訊錄失敗處理
function err_roster(e){
//alert(e.toString());
$("#contact_ul").append("<p>通訊錄取得失敗...</p>");
}
//選擇通訊對象
function choose_receiver(jid){
var jid_ary = jid.split("@");
if(jid_ary.length > 1){
my.receiver = jid;
}else if(jid_ary.length === 1){
my.receiver = jid+"@"+SHORT_HOST_NAME;
}else{
my.receiver = LOGON_USER+"@"+SHORT_HOST_NAME;
}
$("#chat_title").empty();
$("#chat_title").append("<p>目前聊天對象:"+my.receiver+"</p>");
}
|