互動式網頁中有兩個常見功能
1.在主畫面跳出新的表單輸入視窗
2.點圖放大
原畫面會變黑,並由新的視窗覆蓋在原本主要畫面上,這種效果可以用Bootstrap的Modal來完成。以下就用兩個範例來示範(ASP.Net MVC + AngularJS)
互動式網頁中有兩個常見功能
1.在主畫面跳出新的表單輸入視窗
2.點圖放大
原畫面會變黑,並由新的視窗覆蓋在原本主要畫面上,這種效果可以用Bootstrap的Modal來完成。以下就用兩個範例來示範(ASP.Net MVC + AngularJS)
*相關進階設定請參考:
程式前沿-Bootstrap每天必學之模態框(Modal)外掛
這兩篇說明比較詳細,本範例只是濃縮過後的精簡版
範例1,在主畫面跳出新的表單輸入視窗:
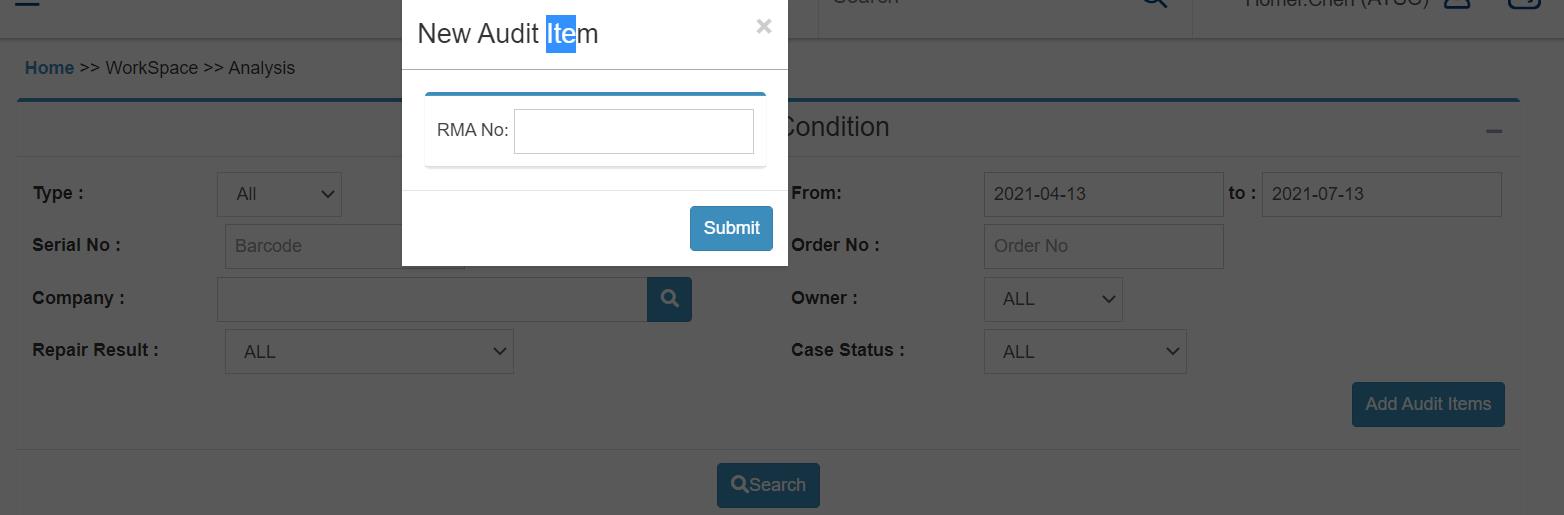
1.前端畫面設定如下(寫成PartialView),使用模態框的彈窗元件需要先設定三層 div 容器元素,分別為 modal(模態宣告層)、dialog(視窗宣告層)、content(內容層)。在內容層裡面,還有三層,分別為 header(頭部)、body(主體,新表單主要內容都是寫在這裡面)、footer(註腳)。因為是搭配AngularJS,所以要控制新視窗的元件的話,要設定ng-model屬性
<!-- 模態宣告,show 表示顯示 -->
<div class="modal" tabindex="-1" id="addnewitem">
<!-- 視窗宣告 -->
<div class="modal-dialog">
<!-- 內容宣告 -->
<div class="modal-content">
<!-- 頭部 -->
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">
<span>×</span>
</button>
<h4 class="modal-title">New Audit Item</h4>
</div>
<!-- 主體 -->
<div class="modal-body">
<div class="form-inline box box-primary" style="margin-bottom:0px;">
<div class="box-header with-border">
<div class="form-inline">
@Html.Translation("RMA No"):
<div class="input-group">
<input id="rma_no" type="text" ng-model="data.searchresult.Order_No" placeholder="" class="form-control" />
</div>
</div>
</div>
</div>
</div>
<!-- 註腳 -->
<div class="modal-footer">
<button id="save_erma_item" type="button" data-loading-text="<i class='fa fa-spinner fa-spin'></i> Submit..." ng-click="save_erma_item_events()" class="btn btn-primary">Submit</button>
</div>
</div>
</div>
</div>
2.回到主要index.cshtml(主畫面),記得把步驟1.的PartialView Render到主要畫面,不然到最後怎麼測試都不會有Modal效果!!
@Html.Partial("~/Areas/WorkSpace/Views/Analysis/_AddAuditItems.cshtml")
3.因為我們有在新視窗的button元件加上ng-click事件,所以我們要在主要index.cshtml的AngularJS的Controller裡面設定的對應的function內容。以下可知,click事件觸發後主要是透過ajax把使用者輸入的內容post到後端作其他商業邏輯處理,並接收return會來的值再來做後續的彈跳訊息視窗顯示
$scope.save_erma_item_events = function () {
$.ajax({
url:'@Url.Action("AddAuditItems", "Analysis", new { Area = "WorkSpace"})',
method: 'post',
data: { para: JSON.stringify($scope.data.searchresult) },
dataType: 'text',
success: function (res) {
if (res.length <= 0) {
showDialog("Add Success!", 'notice', 'Notice', null, 1, function () {
$('#addnewitem').modal('hide');
});
}
else {
showDialog("Add Fail!<br>" + res, 'alert', 'Notice', null, 1, function () {
//$('#addnewitem').modal('hide');
});
}
},
error: function (xhr, status, error) {
var err = eval("(" + xhr.responseText + ")");
alert();
showDialog("Add Fail!\r\n" + err.Message, 'alert', 'Notice', null, 1, function () {
});
},
complete: function () {
$('#rma_no').val('');
}
});
}
[HttpPost]
public string AddAuditItems(string para) {
string Res = "";
JObject obj = JObject.Parse(para);
if (obj != null)
{
try
{
eRMA.Services.Business.WorkSpace.Analysis Business_Analysis = new eRMA.Services.Business.WorkSpace.Analysis(ERMA_User, eRMADate);
var OrderNo = (string)obj["Order_No"];
Business_Analysis.AddAuditItem(OrderNo);
}
catch (Exception ex)
{
Res = ex.Message;
}
}
return Res;
}
範例2,點圖放大效果:
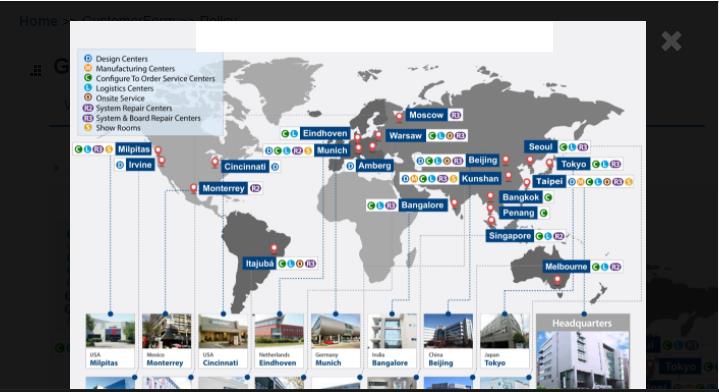
先在index.cshtml分別加上img元件(用在主畫面顯示的縮圖)以及div元容器(用來顯示大圖的model,並把class的show拿掉,預設不顯示)
<img id="myImg" src="~/Images/home/AGS-Map-w2264px.jpg" width="722" height="532">
<!-- The Modal -->
<div id="myModal" class="modal">
<span class="close">×</span>
<img class="modal-content" id="img01">
<div id="caption"></div>
</div>
css:
#myImg {
border-radius: 5px;
cursor: pointer;
transition: 0.3s;
}
#myImg:hover {opacity: 0.7;}
/* The Modal (background) */
.modal {
display: none; /* Hidden by default */
position: fixed; /* Stay in place */
z-index: 1; /* Sit on top */
padding-top: 100px; /* Location of the box */
left: 0;
top: 0;
width: 100%; /* Full width */
height: 100%; /* Full height */
overflow: auto; /* Enable scroll if needed */
background-color: rgb(0,0,0); /* Fallback color */
background-color: rgba(0,0,0,0.9); /* Black w/ opacity */
}
/* Modal Content (image) */
.modal-content {
margin: auto;
display: block;
width: 80%;
/*max-width: 700px;*/
}
/* Caption of Modal Image */
#caption {
margin: auto;
display: block;
width: 80%;
max-width: 700px;
text-align: center;
color: #ccc;
padding: 10px 0;
height: 150px;
}
/* Add Animation */
.modal-content, #caption {
-webkit-animation-name: zoom;
-webkit-animation-duration: 0.6s;
animation-name: zoom;
animation-duration: 0.6s;
}
@@-webkit-keyframes zoom {
from {-webkit-transform:scale(0)}
to {-webkit-transform:scale(1)}
}
@@keyframes zoom {
from {transform:scale(0)}
to {transform:scale(1)}
}
/* The Close Button */
.close {
position: absolute;
/* top: 15px;*/
right: 35px;
color: #f1f1f1;
font-size: 40px;
font-weight: bold;
transition: 0.3s;
}
.close:hover,
.close:focus {
color: #bbb;
text-decoration: none;
cursor: pointer;
}
/* 100% Image Width on Smaller Screens */
@@media only screen and (max-width: 700px){
.modal-content {
width: 100%;
}
}