利用ManualResetEvent來控制子執行緒進行同步作業
using System;
using System.Threading;
public class Example
{
// mre is used to block and release threads manually. It is
// created in the unsignaled state.
private static ManualResetEvent mre = new ManualResetEvent(false);
static void Main()
{
Console.WriteLine("\nStart 3 named threads that block on a ManualResetEvent:\n");
for (int i = 0; i <= 2; i++)
{
Thread t = new Thread(ThreadProc);
t.Name = "Thread_" + i;
t.Start(); //開始線程
}
Thread.Sleep(500);
Console.WriteLine("\nWhen all three threads have started, press Enter to call Set()" +
"\nto release all the threads.\n");
Console.ReadLine();
mre.Set();
Thread.Sleep(500);
Console.WriteLine("\nWhen a ManualResetEvent is signaled, threads that call WaitOne()" +
"\ndo not block. Press Enter to show this.\n");
Console.ReadLine();
for (int i = 3; i <= 4; i++)
{
Thread t = new Thread(ThreadProc);
t.Name = "Thread_" + i;
t.Start();
}
Thread.Sleep(500);
Console.WriteLine("\nPress Enter to call Reset(), so that threads once again block" +
"\nwhen they call WaitOne().\n");
Console.ReadLine();
mre.Reset();//這行之前的執行緒(0~4)都還是為Set()。之後的Thread_5才會為Reset(),又恢復不會執行WaitOne後面的code
// Start a thread that waits on the ManualResetEvent.
Thread t5 = new Thread(ThreadProc);
t5.Name = "Thread_5";
t5.Start();
Thread.Sleep(500);
Console.WriteLine("\nPress Enter to call Set() and conclude the demo.");
Console.ReadLine();
mre.Set();
// If you run this example in Visual Studio, uncomment the following line:
//Console.ReadLine();
}
private static void ThreadProc()
{
string name = Thread.CurrentThread.Name;
Console.WriteLine(name + " starts and calls mre.WaitOne()");
mre.WaitOne(); //阻塞線程,直到調用Set方法才能繼續執行
Console.WriteLine(name + " ends.");
}
}
執行結果:
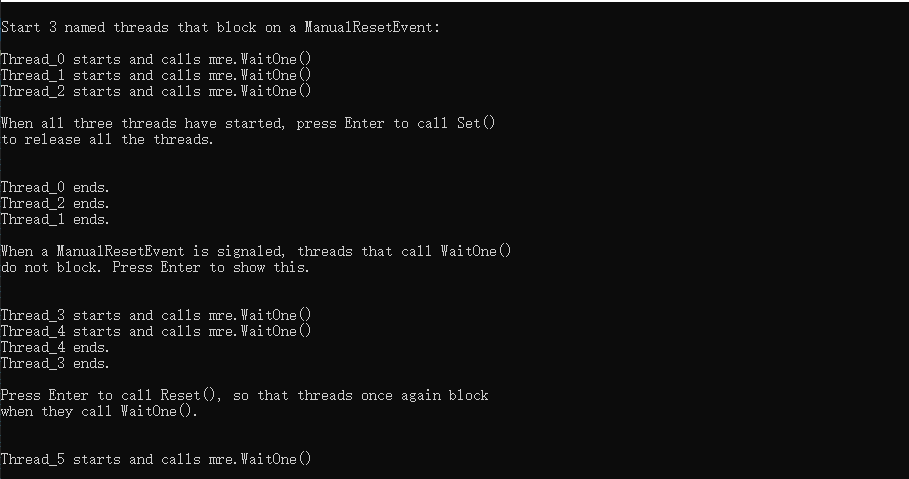
MRE有3種控制執行緒狀態的方法:
1.Set()=>關閉WaitOne訊號,(ThreadPool撈出的)執行緒可以繼續執行.WaitOne()之後的程式
2.Reset()=>開啟WaitOne訊號,(ThreadPool撈出的)執行緒遇到WaitOne()會被中止
3.WaitOne()=>MRE設定執行緒中止點,中斷執行緒到重新呼叫Set()。執行緒才會繼續往下執行WaitOne()後面的程式
ManualResetEvent(false) 的建構子參數可以設定初始訊號:
true=>有訊號,預設MRE狀態為Set(),WaitOne失效,可以想像成瑪莉歐吃到無敵星星一樣,遇到WaitOne都不會被阻擋,會往下執行,直到重新呼叫ReSet()時WaitOne()才會重新生效。
false=>無訊號,預設MRE狀態為ReSet(),WaitOne生效()。遇到WaitOne()後執行緒會中止,不會往下執行WaitOne()後的程式,直到重新呼叫Set()時訊號才會變成true,繼續往下執行WaitOne後的程式。如果要把訊號再變為無訊號(false),則要再呼叫Reset(),不然Set()後,Reset()前,WaitOne()會是一直沒用的狀態了。
reference:
ManualResetEvent 類別 = >Microsoft api手冊
C# 執行緒(Thread)的AutoResetEvent及ManualResetEvent探討 =>想了解AutoResetEvent & ManualResetEvent差別可以看這篇
C#使用AutoResetEvent實現同步